React: Babel 7 support in boilerplate application
Introduction
Babel 7 comes after more than 2 years of active development and four thousand commits. The main advantage of the new version is the speed optimizations and configuration flexibility. In this tutorial we’ll see how to upgrade our React boilerplate application to support Babel 7.
Bebel dependencies
The first step in order to support Babel 7 is to replace our old dependencies in package.json
:
1"devDependencies": {
2 "babel-core": "^6.26.3",
3 "babel-loader": "^7.1.4",
4 "babel-preset-env": "^1.7.0",
5 "babel-preset-react": "^6.24.1",
6...
7 },
with new ones:
1"devDependencies": {
2 "@babel/core": "7.2.2",
3 "@babel/plugin-proposal-object-rest-spread": "7.3.2",
4 "@babel/plugin-syntax-dynamic-import": "7.2.0",
5 "@babel/plugin-transform-runtime": "7.2.0",
6 "@babel/preset-env": "7.3.1",
7 "@babel/preset-react": "7.0.0",
8 "@babel/runtime": "7.3.1",
9 "babel-loader": "^8.0.5",
10...
11 },
The main change compared to the older version is Babel’s switch to scoped packages. To summarize, from now on most of the packages named with the prefix babel-
will now be named @babel/
.
Along with the updated dependencies, we’ve added a couple of Bebel plugins in order to support additional ES6 language features
Babel configuration
Next step will be to replace our .babelrc
with babel.config.js
. You can read more about why it’s recommended to use a project wide babel.config.js
here.
The configuration file will be used to load the presets and the plugins we’ve added in our devDependencies section:
1module.exports = {
2 presets: ['@babel/preset-env', '@babel/preset-react'],
3 plugins: [
4 '@babel/plugin-transform-runtime',
5 '@babel/plugin-proposal-object-rest-spread',
6 '@babel/plugin-syntax-dynamic-import'
7 ]
8};
Conclusion
This post is a follow up on the previous post where we prepared a ReactJS application from scratch in order to use Babel 7.
The full source code for this post can be found at Github.
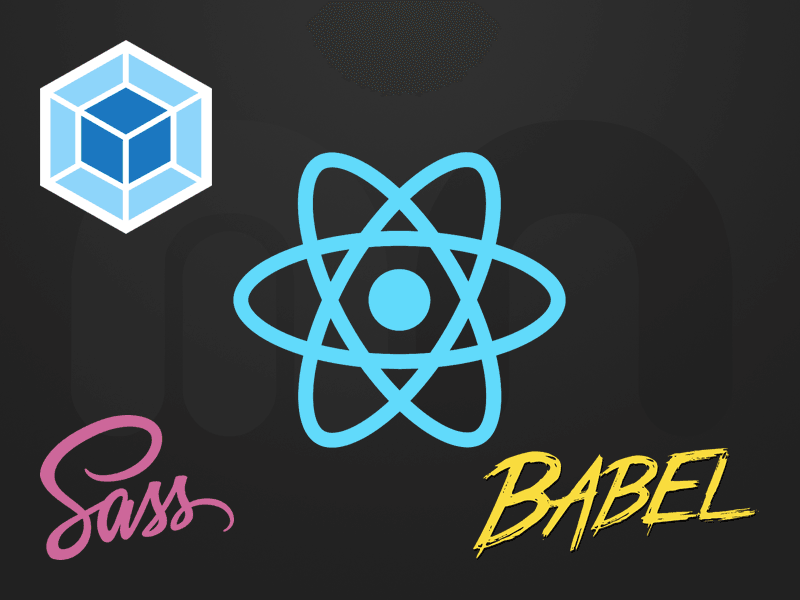