JBang: Unlocking Java's Scripting Potential
Introduction
Java is a powerful language, but traditionally it hasn't been known for rapid scripting or prototyping. In fact, Java's verbosity and boilerplate code have often been cited as reasons why developers turn to other languages for scripting tasks or even smaller applications.
JBang is a tool that aims to change that perception by making Java a first-class citizen in the scripting world. It is a tool designed to let you write, run, and share Java scripts with minimal setup.
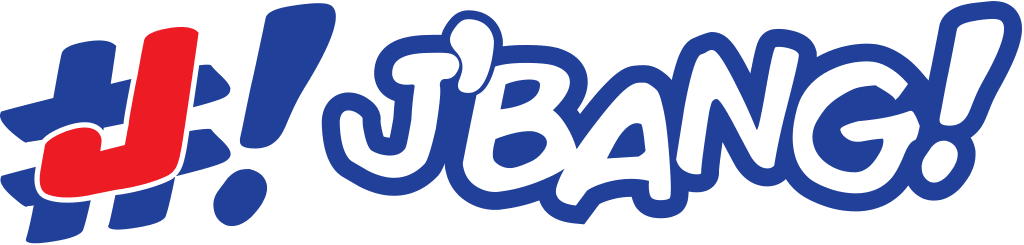
In this post, I'll give you an overview of JBang and show you how to get started with it today.
What is JBang?
JBang is a revolutionary command-line tool that transforms Java into a scripting language, making it as accessible as Python or Shell scripting. It allows developers to create, edit, and run self-contained Java programs with minimal setup, no need for full project setup or cumbersome build files. By eliminating the need for traditional build tools like Maven or Gradle, JBang simplifies Java development, enabling experimentation and quick prototyping. Whether you're writing a quick one-off script or prototyping an idea, JBang allows you to work in plain Java syntax while automatically managing dependencies and even JDK versions when needed.
With JBang, you can:
- Run Java code directly from
.java
files or even from URLs. - Manage dependencies inline using simple comment directives or annotations.
- Debug and edit scripts seamlessly in popular IDEs like IntelliJ IDEA.
Its tagline, "Unleash the power of Java" perfectly encapsulates its mission to make Java more approachable for scripting and lightweight tasks.
Why use JBang?
For years, Java developers have grappled with the lack of a simple scripting solution. While tools like JShell exist, they don't fully address the need for dependency management or script portability.
JBang fills this gap with features such as:
- Ease of Use: You can write a standalone
.java
file with a main method (or even use JShell-style scripts) and run it immediately. - Dependency Management: JBang automatically resolves dependencies from Maven Central or other repositories, making it easy to include libraries in your scripts.
You can add dependencies directly in your source with special comments (e.g.,
//DEPS ...
), and JBang handles the rest. - Portability: It works on Windows, macOS, and Linux. It is loyal to the motto "Write once, run anywhere."
- JDK Bootstrapping: You don't have Java installed? No problem! JBang can download and install the required JDK for you.
- Integration with modern tools: JBang is compatible with popular frameworks and libraries like Quarkus, Spring, and Micronaut. You can even run JBang scripts in GitHub Actions or GitLab CI/CD pipelines.
Getting started with JBang
To get started with JBang, you need to install the tool on your system.
Installation
Installing JBang is straightforward. You can use a single command to set it up:
- Linux/macOS/AIX/WSL bash:
curl -Ls https://sh.jbang.dev | bash -s - app setup
- Windows PowerShell:
iex "& { $(iwr -useb https://ps.jbang.dev) } app setup
- SDKMan:
sdk install jbang
Important
Consider verifying the script's source and checksum before execution for security best practices.
Alternatively, you can download the JBang binaries for your system directly from the website. JBang also supports installation via package managers like Homebrew on macOS or Chocolatey on Windows.
After installation, you can run jbang --version
to verify that JBang is correctly installed.
Writing your first script
Let's create a simple "Hello, World!" script using JBang.
1. Initialize the script
JBang provides a simple way to create a new script by running the following command:
jbang init HelloWorld.java
This creates a file named HelloWorld.java
with a basic template for a JBang script.
The following snippet contains its contents:
///usr/bin/env jbang "$0" "$@" ; exit $?
import static java.lang.System.*;
public class HelloWorld {
public static void main(String... args) {
out.println("Hello World");
}
}
2. Run the script
To run the script, execute the following command:
jbang HelloWorld.java
You should see the output Hello World
in your terminal.
JBang comments or directives
JBang scripts can include special comments or directives that provide additional information to the JBang runtime.
If we examine the HelloWorld.java
script, we see the following comment at the beginning:
///usr/bin/env jbang "$0" "$@" ; exit $?
This is a common shebang that tells the system to run the script with JBang.
If your system is compatible with shebangs, you can run the script directly from the command line without specifying jbang
explicitly.
There many are other directives you can use in JBang scripts, such as:
Directive | Description |
---|---|
//DEPS | Add dependencies to your script.//DEPS org.apache.commons:commons-lang3:3.12.0 |
//SOURCES | Include additional source files.//SOURCES src/main/java/MyUtils.java |
//FILES | Include additional files.//FILES src/main/resources/config.properties |
//JAVA | Specify the Java version to use.//JAVA 11 |
//COMPILE_OPTIONS | Set compiler options.//COMPILE_OPTIONS --enable-preview |
These are some of the most common directives in JBang scripts. However, many more are available, and you can find the complete list in the JBang documentation.
Conclusion
JBang bridges the gap between traditional Java development and the rapid scripting seen in languages like Python or Bash. Whether you're automating tasks, experimenting with new libraries, or teaching Java to beginners, JBang removes the traditional barriers associated with Java development.
Give it a try and see how JBang can streamline your Java development workflow!