Updated: Displaying a jTable inside another jTable // JTable cellRenderer
A few of the blog readers have asked some questions about my previous post. So I've decided to update the code to remove some bugs and post it here.
This is the revised version. Just like in the previous iteration, let's start defining some variables to hold the data for our example's model:
// We initialize the Objects where we'll store our data
// First an array Object which will be our main table
Object[][] data = null;
// We create an Array String to hold the name of the main table columns
//In this example case we are going to store a contact name,
//his email address(es) and his phone numer(s)
//We're also going to store the creation date.
String[] columns = {"Name", "E-Mail","Phone","Creation Date"};
Next, let's create a function to populate the variables with some sample data:
public void populateData() {
// Two contacts
data = new Object[3][columns.length];
data[0][0] = "Peter";
String[] emails = {"peter@yahoo.com", "strange@name.com"};
data[0][1] = emails;
String[] phones = {"555 35 25 65" , "555 35 24 63"};
data[0][2] = phones;
data[0][3] = new Date();
data[1][0] = "Jackson";
data[1][1] = "Jack@hotmail.com";
String[] phones2 = {"555 35 24 33" , "555 11 88 88", "332 55 25 34"};
data[1][2] = phones2;
data[1][3] = new Date();
data[2][0] = "Robert";
data[2][1] = "rob@hotmail.com";
data[2][2] = "555 28 95 81";
data[2][3] = new Date();
// As you can see, we've stored two contacts one with two e-mail
// accounts and two phone numbers, and another member with three phone numbers
}
Finally, we create our table models and the cell renderer that will allow us to display the data in a nested JTable
:
// Next we create our table models
public void createModel(){
/* First we create the main model We overide the AbstractTableModel necessary methods*/
AbstractTableModel modelo = new AbstractTableModel() {
public String getColumnName(int col) {
return columns[col];
}
public Class getColumnClass(int col) {
if(getRowCount() <1) {
return null;
}
return data[0][col].getClass();
}
public int getRowCount() {
return data.length;
}
public int getColumnCount() {
return columns.length;
}
public Object getValueAt(int row, int col) {
return data[row][col];
}
public boolean isCellEditable(int row, int col){
return true;
}
public void setValueAt(Object value, int row, int col) {
data[row][col] = value;
fireTableCellUpdated(row, col);
}
};
// We apply the model to the main jTable
jTableData.setModel(modelo);
// We create a cell Renderer to display the data of the multivalue fields
TableCellRenderer jTableCellRenderer = new TableCellRenderer() {
/* Magic Happens */
public Component getTableCellRendererComponent(
JTable table, Object value, boolean isSelected, boolean hasFocus, int row, int column) {
/* If what we're displaying isn't an array of values we return the normal renderer*/
if(!value.getClass().isArray()){
return table.getDefaultRenderer(value.getClass())
.getTableCellRendererComponent( table, value, isSelected, hasFocus,row, column);
} else {
final Object[] passed = (Object[])value;
// We create the table that will hold the multivalue
// fields and that will be embedded in the main table
JTable embedded = new JTable(new AbstractTableModel() {
public int getColumnCount() {
return 1;
}
public int getRowCount() {
return passed.length;
}
public Object getValueAt(int rowIndex, int columnIndex) {
return passed[rowIndex];
}
public boolean isCellEditable(int row, int col){
return true;
}
});
if(isSelected){
embedded.setBackground(jTableData.getSelectionBackground());
embedded.setForeground(jTableData.getSelectionForeground());
}
if(hasFocus){
embedded.setRowSelectionInterval(0,1);
}
// If this is what you plan to enable mouseClick detection,
// in your table, IT WONT WORK. Have a look at TableCellEditor.
embedded.addMouseListener(new MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
System.out.println("PEPE");
}
});
setPreferredSize(embedded.getPreferredSize());
if(getPreferredSize().height != table.getRowHeight(row)) {
table.setRowHeight(row, getPreferredSize().height);
}
return embedded;
}
}
};
// Finally we apply the new cellRenderer to the whole table
TableColumnModel tcm = jTableData.getColumnModel();
for(int it = 0; it < tcm.getColumnCount(); it++){
tcm.getColumn(it).setCellRenderer(jTableCellRenderer);
// tcm.getColumn(it).setCellEditor(jTableCellEditor);
}
// Note: if we need to edit the values inside the embedded jtable
// we will need to create a TableCellEditor too.
}
If everything goes well, you should see a table like the following:
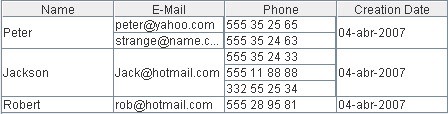
Comments in "Updated: Displaying a jTable inside another jTable // JTable cellRenderer"
Second, you CAN get events to the inner JTable. I keep the JTables around, and if the embedded.MouseListener sees it's been clicked on a cell with a JTable, it does a 'dispatchEvent' on the sub-table handing it the event.
Now, if I could just get highlighting/selection to work properly...
What you say its true, you can make your upper table dispatch events to your subsequent jTables. But although you can achieve what you want (partly) its NOT a recommended way of doing things.
TableCellRenderer doesn't build a component for each cell in your JTable, that would be very memory consuming. So if you want to dispatch events to the inner JTables, you must do as you say "keep them around". The correct/elegant/performance way of doing this is implementing your own TableCellEditor.
Further reference can be found in this link:
Sun Bug Database 4136681
but i am facing one proble ..
if i put JTable inside JTable cell....it's not showing whole table i mean it's not showing inside table's column how to do that....plz help me if possible....
besides that, i was having trouble trying to read that data from a list and asiging it to the String[] or to the Data[][]... any ideas?
Thank you, the code is perfectly working for me.
If I try to copy the table data, the embedded cell values are copied as an Object
i.e. Peter [Ljava.lang.Object;@20785a37 [Ljava.lang.Object;@2d649736 04-abr-2007
How can i copy the data as it is for embedded cells instead of Objects.