Kubernetes Client for Java: How to set up the underlying HTTP client
Introduction
The Fabric8 Kubernetes Client stands out as one of the most popular Java clients for Kubernetes. If you compare it to other clients, it has an additional rich set of features and performance optimizations that set it apart from other Kubernetes clients.
One remarkable feature introduced in version 6.0 allows you to configure the underlying HTTP client used by the Kubernetes client. This unique capability makes the Fabric8 Client an incredibly flexible choice.
Choosing the right HTTP client is particularly valuable when you want to align your Kubernetes client with the HTTP client already in use by your application. For instance, the Fabric8 Kubernetes Client instance provided by Quarkus defaults to Eclipse Vert.x.
The Fabric8 Kubernetes client offers multiple built-in HTTP client implementations. You will likely find one that fits your needs. Let's explore these options in detail and learn how to configure them.
Available HTTP client implementations
Currently, the Fabric8 Kubernetes Client provides the following HTTP client implementations:
OkHttp is the default HTTP client implementation provided by Fabric8.
This has been traditionally the case since the first versions of the client.
This is also the main reason why it's still shipped by default and why it's provided as a transitive dependency
of the kubernetes-client
module.
Whenever we add a dependency to a different HTTP client implementation, this one will take precedence over OkHttp.
However, it's recommended that we exclude the kubernetes-httpclient-okhttp
dependency to improve our project build performance.
Let us now continue by learning how to configure the vanilla JDK HTTP client.
How to configure the vanilla Java JDK HttpClient
Starting from Java 11, the JDK provides a built-in HTTP client. This is extremely convenient because nowadays, most applications need to communicate with external services and applications through HTTP.
From the Java Kubernetes Client perspective, being able to rely on an HTTP client without adding any additional dependencies is a great advantage. When we released version 6.0, we considered shipping the vanilla JDK HTTP client as the default implementation. However, the JDK HTTP client has some issues that weren't fixed until Java 16. You can find more information about this in the module's readme.
In any case, if you're using Java 16 or any of the new LTS versions, you can safely configure the Kubernetes client to use the JDK HTTP client.
The following snippet shows how to set up your project's pom.xml
file to use the JDK HTTP client:
<project>
<dependencies>
<dependency>
<groupId>io.fabric8</groupId>
<artifactId>kubernetes-client</artifactId>
<exclusions>
<exclusion>
<groupId>io.fabric8</groupId>
<artifactId>kubernetes-httpclient-okhttp</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>io.fabric8</groupId>
<artifactId>kubernetes-httpclient-jdk</artifactId>
</dependency>
</dependencies>
</project>
Or in case you're using Gradle:
dependencies {
implementation("io.fabric8:kubernetes-client") {
exclude group: "io.fabric8", module: "kubernetes-httpclient-okhttp"
}
implementation("io.fabric8:kubernetes-httpclient-jetty")
}
Let's now continue by learning how to configure the Eclipse Vert.x HTTP client.
How to configure Eclipse Vert.x
Eclipse Vert.x is a toolkit for building reactive applications on the JVM. It also provides a non-blocking HTTP client that can be used to communicate with external services and applications. Since this is one of the most performant clients available, it's a great choice for the Kubernetes client.
The following code snippet shows how to configure the Kubernetes client to use the Vert.x HTTP client:
<project>
<dependencies>
<dependency>
<groupId>io.fabric8</groupId>
<artifactId>kubernetes-client</artifactId>
<exclusions>
<exclusion>
<groupId>io.fabric8</groupId>
<artifactId>kubernetes-httpclient-okhttp</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>io.fabric8</groupId>
<artifactId>kubernetes-httpclient-vertx</artifactId>
</dependency>
</dependencies>
</project>
Or in case you're using Gradle:
dependencies {
implementation("io.fabric8:kubernetes-client") {
exclude group: "io.fabric8", module: "kubernetes-httpclient-okhttp"
}
implementation("io.fabric8:kubernetes-httpclient-vertx")
}
How to configure Eclipse Jetty
Eclipse Jetty is mainly a Java HTTP server and servlet container. However, Jetty also provides a high-performance HTTP client that can be used to communicate with external services and applications.
Jetty is also a good choice in case your project is using JDK 11 or a more recent version. The following code snippet shows how to configure the Kubernetes client to use the Jetty HTTP client:
<project>
<dependencies>
<dependency>
<groupId>io.fabric8</groupId>
<artifactId>kubernetes-client</artifactId>
<exclusions>
<exclusion>
<groupId>io.fabric8</groupId>
<artifactId>kubernetes-httpclient-okhttp</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>io.fabric8</groupId>
<artifactId>kubernetes-httpclient-jetty</artifactId>
</dependency>
</dependencies>
</project>
Or in case you're using Gradle:
dependencies {
implementation("io.fabric8:kubernetes-client") {
exclude group: "io.fabric8", module: "kubernetes-httpclient-okhttp"
}
implementation("io.fabric8:kubernetes-httpclient-jetty")
}
As we've learned, the Fabric8 Kubernetes Client provides multiple HTTP client implementations out of the box. Let us now see what to do in case you want to use a different HTTP client.
How can I use XXX HTTP client?
In case the HTTP client you want to use is not provided by the Fabric8 Kubernetes Client,
you can still use it by implementing theHttpClient
interfaces.
Despite this not being a very easy task, it's still possible to do it.
For example, the Armeria folks are already working on an
implementation of the HttpClient
interface.
Conclusion
In this article, I've shown you how to configure the Fabric8 Kubernetes Client to use different underlying HTTP clients. Being able to choose the HTTP client is a great feature that allows you to use the same HTTP client that your application may already be using.
The Fabric8 Kubernetes Client already provides multiple HTTP client implementations out of the box.
We've also learned that you can implement the HttpClient
interface in case you want to use a different HTTP client.
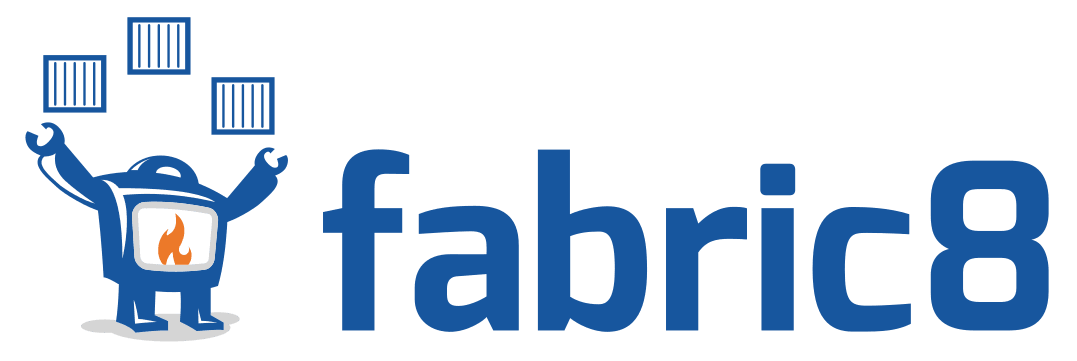