Kubernetes Client for Java: Fabric8 introduction
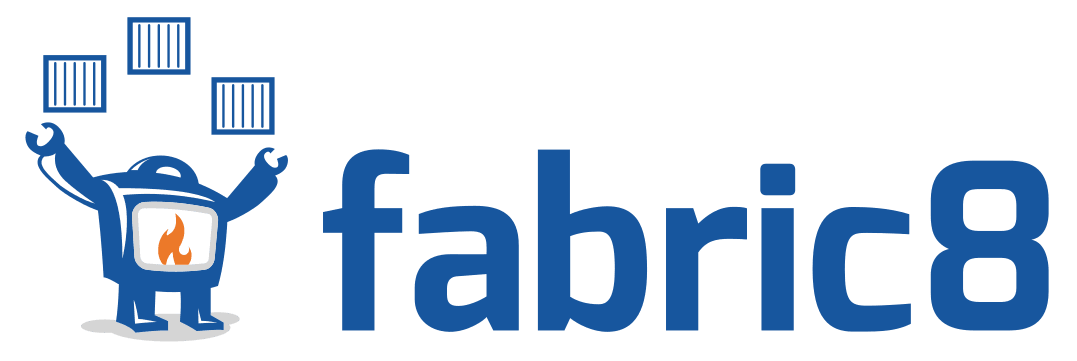
Introduction
The Kubernetes Java world is a very rich ecosystem with an endless amount of resources. For instance, when you need to interact with the Kubernetes API server, you have a few options to choose from.
In an earlier post I introduced you to YAKC, a client I implemented as a side project. Today I'm going to talk about the other client I officially maintain, the Fabric8 Kubernetes Client.
The Fabric8 Kubernetes Client is the oldest Java Kubernetes Client of the bunch. And even though there is now an official CNCF Java Client for Kubernetes, Fabric8 remains one of the most popular options.
The Fabric8 client differentiates itself from the rest because it provides a fluent DSL. Both, to perform operations, and to instantiate and modify resource types.
What are the main components of the Fabric8 Kubernetes Client?
Cluster specific implementations
Depending on the cluster type you are targeting, there are different options you can choose from. The basic choice is the kubernetes-client
artifact, which should be compatible with any Kubernetes implementation (including OpenShift). However, if you want to take full advantage of OpenShift and its unique resources, you should use the more specific artifact openshift-client
.
Cluster | Component/Artifact |
---|---|
Kubernetes | kubernetes-client |
OpenShift | openshift-client |
Available Extensions
In addition to the base client, there is a growing number of extensions available as part of the Fabric8 Kubernetes Client distribution.
How do I set up Fabric8 Kubernetes Client in my project?
The easiest way to get started is by including your required component dependency in your project.
If you are using Maven, this can be as easy as:
<dependency>
<groupId>io.fabric8</groupId>
<artifactId>kubernetes-client</artifactId>
<version>6.13.0</version>
</dependency>
Or if your project is based on Gradle:
dependencies {
implementation 'io.fabric8:kubernetes-client:6.13.0'
}
Operation overview
The Fabric8 Java Client should allow you to perform at least the same operations you can execute with Kubectl or the Kubernetes Go client.
In the following sections, you will find a summary of the most common tasks (usually CRUD related) that you can achieve while using the Fabric8 client.
Retrieve a list of resources
The next example returns a list of Pods
for the specified namespace:
try (KubernetesClient kc = new KubernetesClientBuilder().build()) {
kc.pods().inNamespace("my-namespace").list().getItems()
.forEach(pod ->
System.out.printf("Pod %s %n", pod.getMetadata().getName()));
}
Retrieve a resource with a given name
The following example retrieves a Pod
with the provided name from the specified namespace:
Pod pod = kc.pods().inNamespace("my-namespace").withName("my-pod").get();
Create a resource
The hereunder example creates a ConfigMap
in the specified namespace:
kc.configMaps().inNamespace("my-namespace").create(new ConfigMapBuilder()
.withNewMetadata().withName("my-configmap").endMetadata()
.addToData("data-field", "data-value")
.build()
);
Edit a resource with a given name
The following example adds an annotation to a Pod
with the provided name from the specified namespace:
kc.pods().inNamespace("my-namespace").withName("my-pod")
.edit(p -> new PodBuilder(p)
.editMetadata().addToAnnotations("edited", "true").endMetadata().build());
Delete a named resource
The example below deletes a Pod
with the provided name in the specified namespace and waits 10 seconds for the operation to complete:
kc.pods().inNamespace("my-namespace").withName("my-pod").delete();
kc.pods().inNamespace("my-namespace").withName("my-pod")
.waitUntilCondition(Objects::isNull, 10, TimeUnit.SECONDS);
These are just a few examples that showcase some of the many features that you'll get if you choose to use the Fabric8 Kubernetes Client. You can find many more examples in the official Cheatsheet.
Conclusion
In this post, I gave you a very light introduction to the Fabric8 Kubernetes Client and its components. I also included some code snippets describing how to perform basic operations in your cluster.
I will publish further posts with more detailed instructions for more involved operations. There are also complementary components in the suite, such as the Mock Server, that I'd like to showcase in a dedicated post.
In the meantime, you can learn more about the client by checking the following resources: