Viewing and storing images from an IP Camera
So you bought an IP camera and would like to store/see images from the camera using Java. Recently I bought a couple of IP Cameras for testing purposes. I noticed that each IP camera has different surveillance tools and web interfaces. But most of them have in common that you can access the actual picture by entering an URL in your browser.
In this post I'll show you how to see that image and store it using a very simple Java application. The camera I'll use for the test is a LinkSys WVC200 "Wireless PTZ Internet Camera with Audio". This is the cheapest wireless IPcam I found with at least some decent features.
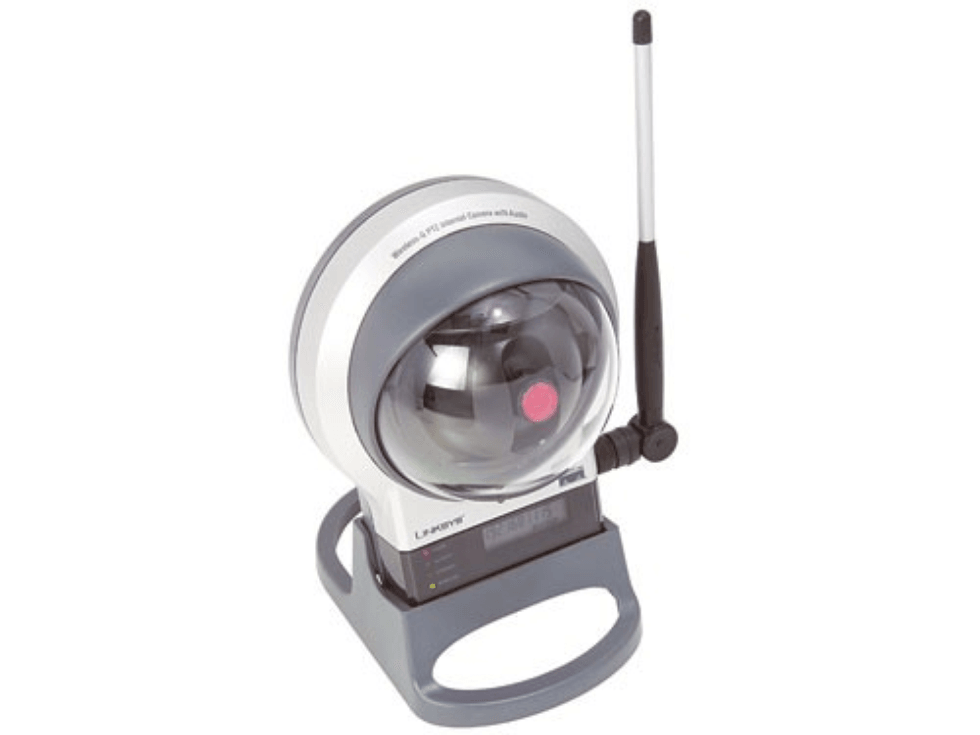
This camera has a nice web interface, but for visualization purposes, you will need to be sitting in front of a windows desktop. This camera, as many others, uses an ActiveX control to show/control the image. However, LinkSys is kind enough to offer the camera's Firmware source code.
If you analyze the source code you can find some clues to get the URL where the static image is served (there's an URL for ASF video format too, with some time you can develop an app to store and play the video). For this camera, the URL is http://CAMERAIP/img/snapshot.cgi?size=640x480"
.
With this information, we can build an application to grab the image and show/store the image. If you have another type of camera, you can adapt the program by specifying the URL for that camera.
1try {
2 // Build a new URL specifying the path to where the image
3 // is served
4 URL url = new URL ( "http://192.168.10.80/img/snapshot.cgi?size=640x480");
5 // String with the user and password to access the camera interface
6 // (userName and password that you would enter in the browser
7 String userPassword = "USER:PASSWORD";
8 // We must encode with Base64 to supply it with the header.
9 String encoding = new Base64Encoder(userPassword).processString();
10 // Open the connection
11 URLConnection uc = url.openConnection();
12 // Specify the authorization
13 uc.setRequestProperty ("Authorization", "Basic " + encoding);
14 // Load a copy of the image into memory
15 BufferedImage img = ImageIO.read(uc.getInputStream());
16 // Show the image in a JLabel
17 jLImagen.setIcon(new ImageIcon(img));
18 // Store the image in the specified path
19 ImageIO.write(img, "jpg", new File("pathToFile"));
20} catch (MalformedURLException ex) {
21 ex.printStackTrace();
22} catch (IOException ex) {
23 ex.printStackTrace();
24}
Extending this simple application by adding some functionality can bring a very good surveillance application. If you analyze most commercial surveillance applications to use with different ipCameras you'll notice that although they are very expensive the core of the application is similar to the 10 lines of code above.
Comments in "Viewing and storing images from an IP Camera"
the above code?
Can you get 15fps?
Thanks.
The problem with the above code is that each time you get a photo you must open a new connection/request which takes some time, depending on the network (but even with good Intranets, it's slow). It would be the same as pressing the refresh [F5] button in your browser. This is why most cameras offer another type of stream, normally an mjpg (aka many jpg images joint together in one file). If you need such a quick refresh rate (15 fps) you can study capturing the mjpg stream and then separating each jpg from the stream. It's more complicated but possible.
To do this you should analayze the input stream and search for the BOMof a jpg then save that part of the stream to a separate jpg file.
You can also try to find a library that can process mjpg or any other type of stream.
Regards
Many thanks for the code!
I have only two issues(still):
String encoding = new Base64Encoder(userPassword).processString();
cannot find Base64Encoder import?
and JLabel ? jLImagen imports:
can you please advize?
I get the error:
java.io.IOException: Server returned HTTP response code: 401 for URL:...
any ideas why:
BufferedImage img = ImageIO.read(uc.getInputStream());
fails?
thank you