Trigger Kubernetes CronJob manually from Java using YAKC
Introduction
In this post, I’ll show you how to perform the equivalent to kubectl --from=cronjob/<cronjob-name> <job-name>
(manual trigger) from Java using YAKC- Yet Another Kubernetes Client.
This performs something similar to what the official Kubernetes Dashboard CronJob trigger action does.
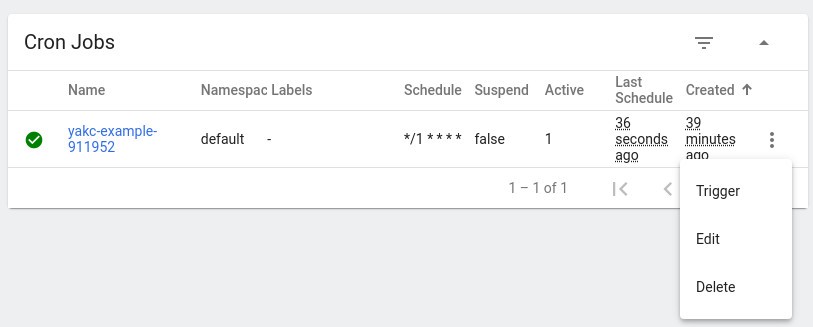
CronJob manual trigger
Once you’ve deployed a CronJob to your cluster, in most cases it makes sense to trigger a test run. You probably need to check that everything works fine without having to wait for the scheduled time.
Starting at version 1.10, Kubernetes offers a way to create a Job from a CronJob using kubectl
. This basically translates into manually triggering the CronJob.
Following is the code implementation in Kubectl:
1func (o *CreateJobOptions) createJobFromCronJob(cronJob *batchv1beta1.CronJob) *batchv1.Job {
2 annotations := make(map[string]string)
3 annotations["cronjob.kubernetes.io/instantiate"] = "manual"
4 for k, v := range cronJob.Spec.JobTemplate.Annotations {
5 annotations[k] = v
6 }
7
8 job := &batchv1.Job{
9 // this is ok because we know exactly how we want to be serialized
10 TypeMeta: metav1.TypeMeta{APIVersion: batchv1.SchemeGroupVersion.String(), Kind: "Job"},
11 ObjectMeta: metav1.ObjectMeta{
12 Name: o.Name,
13 Annotations: annotations,
14 Labels: cronJob.Spec.JobTemplate.Labels,
15 OwnerReferences: []metav1.OwnerReference{
16 {
17 APIVersion: batchv1beta1.SchemeGroupVersion.String(),
18 Kind: cronJob.Kind,
19 Name: cronJob.GetName(),
20 UID: cronJob.GetUID(),
21 },
22 },
23 },
24 Spec: cronJob.Spec.JobTemplate.Spec,
25 }
26 if o.EnforceNamespace {
27 job.Namespace = o.Namespace
28 }
29 return job
30}
In the previous code snippet, we see how kubectl
creates a new Job
using the CronJob’s JobTemplate
and propagates the labels.
Trigger CronJob from Java
Now that we’ve seen how kubectl
creates a Job
from a CronJob
, let’s see how we can achieve this with YAKC.
1final CronJob cronJob = kc.create(BatchV1beta1Api.class).readNamespacedCronJob(name, applicableNamespace).get();
2final String jobName = String.format("%s-manual-%s",
3 name.length() > 38 ? name.substring(0, 38) : name,
4 new Random().nextInt(999999)
5);
6kc.create(BatchV1Api.class).createNamespacedJob(applicableNamespace, Job.builder()
7 .metadata(ObjectMeta.builder()
8 .name(jobName).namespace(applicableNamespace)
9 .labels(new HashMap<>(Optional.ofNullable(cronJob.getMetadata().getLabels()).orElse(Collections.emptyMap())))
10 .putInAnnotations("cronjob.kubernetes.io/instantiate", "manual")
11 .addToOwnerReferences(OwnerReference.builder()
12 .kind(cronJob.getKind())
13 .apiVersion(cronJob.getApiVersion())
14 .controller(false)
15 .name(cronJob.getMetadata().getName())
16 .uid(cronJob.getMetadata().getUid())
17 .build())
18 .build())
19 .spec(cronJob.getSpec().getJobTemplate().getSpec())
The previous code snippet is part of the CronJobs jbang quickstart.
The first step is to retrieve the actual CronJob
from the cluster. Next, we generate a random name for the manually triggered job using the CronJob’s name as the prefix.
Finally, we create a new Job
using the retrieved CronJob’s JobTemplateSpec
and propagate its labels. We also add an owner reference to the created Job so that it can be associated and listed when querying the CronJob
.
Since this example is hosted as a jbang script, you can test it by running the following command:
1jbang https://github.com/manusa/yakc/blob/master/quickstarts/jbang/CronJobs.java trigger $cronJobName
Following you can check the operation result in YAKC – Kubernetes Dashboard interface (you can read more about this project here):
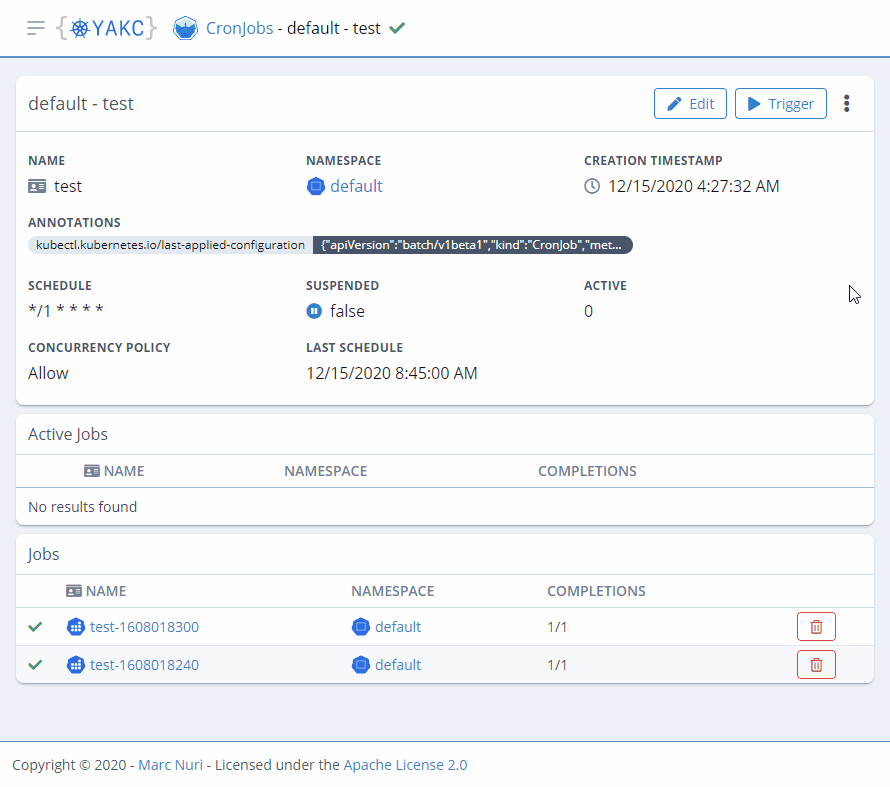
Conclusion
In this post, I’ve shown you how to manually trigger CronJobs from Java using YAKC.
I’ve extracted the code for this post from the jbang quickstart section of YAKC. You can learn more by visiting YAKC’s GitHub project site. You can also see related code here.