Kubernetes 1.19 Ingress API from Java using YAKC
Introduction
Kubernetes v1.19.0 was just released. Amongst the many new features and improvements, the graduation of Ingress API to V1 (#1453) stands out.
Despite users have widely adopted Ingress resources, and that Kubernetes introduced the beta feature back in 2015 (v1.1), it hasn't been until the new v1.19 release that Ingress has gone GA.
An Ingress is “An API object that manages external access to the services in a cluster, typically HTTP”. In other words, Ingresses are the way to publicly expose your Kubernetes managed Services to the outer world.
In this post, I will show you how to use YAKC to create a new Ingress using the new v1 API introduced in Kubernetes v1.19.0.
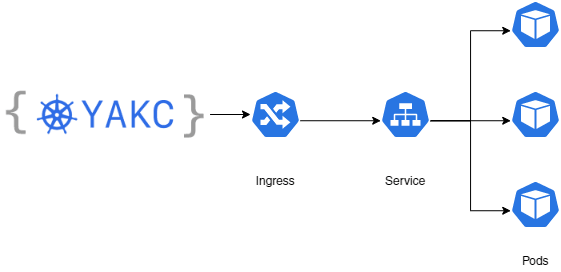
Ingress from Java
You can use Ingresses to provide external reachable URLs, and traffic load balancing to Service resources. In addition, Ingress resources are useful if you want to provide name-based virtual hosting capabilities and SSL termination.
The Ingress resource requires an IngressController to be used. Kubernetes currently officially supports and maintains GCE and NGINX controllers.
Note that Ingress v1
introduces several changes to v1beta1
, most notably, the pathType
field is now required and no longer provides a default value.
The following YAML describes an Ingress
using en existent NGINX IngressController
:
1apiVersion: networking.k8s.io/v1
2kind: Ingress
3metadata:
4 name: ingress-example
5 namespace: default
6spec:
7 ingressClassName: nginx
8 rules:
9 - host: "*.foo.com"
10 http:
11 paths:
12 - path: /
13 pathType: Exact
14 backend:
15 serviceName: path-exact
16 servicePort: 80
You can achieve the exact same result using YAKC:
1new KubernetesClient().create(NetworkingV1Api.class).createNamespacedIngress("default", Ingress.builder()
2 .metadata(ObjectMeta.builder()
3 .name("ingress-example")
4 .build())
5 .spec(IngressSpec.builder()
6 .addToRules(IngressRule.builder()
7 .host("*.foo.com")
8 .http(HTTPIngressRuleValue.builder()
9 .addToPaths(HTTPIngressPath.builder()
10 .path("/")
11 .pathType("Exact")
12 .backend(IngressBackend.builder()
13 .service(IngressServiceBackend.builder()
14 .name("path-exact")
15 .port(ServiceBackendPort.builder()
16 .number(80)
17 .build())
18 .build())
19 .build())
20 .build())
21 .build())
22 .build())
23 .build())
24 .build()
25).get();
The main advantage of using Java (YAKC Kubernetes Client) is that you can perform these tasks dynamically. For instance, you can implement a Java-based Operator that will automatically create an Ingress whenever a Service gets created.
Conclusion
Kubernetes 1.19 marks the general availability of Ingress networking.k8s.io/v1
. In this post, I’ve shown you how to use YAKC to create an Ingress using Java.
You can learn more by visiting YAKC’s GitHub project site. You can also see related code here.