Java 10: Testing the new release
Introduction
Java 10 was released the past 20th of March (2018) following the new tight six-month release schedule. This version comes just after the Java 9 release on September 2017 and marks this past release obsolete. Same will happen to Java 10 on September this year, when the release of Java 11 will mark this one obsolete.
Java 11 will be released as a long term support (LTS) version and will have a much longer lifespan, but until then, let’s take a look at some of the new features in Java 10.
Time-Based Release Versioning
Java 10 introduces the new time-based release versioning (JEP 322) which is a recast of JEP 223 delivered on Java 9 to enable the new release model. From now on version numbers will follow this pattern:
$FEATURE.$INTERIM.$UPDATE.$PATCH
Every six months a new feature version will be released, every three years starting on September 2018 the feature release will be a long-term support release.
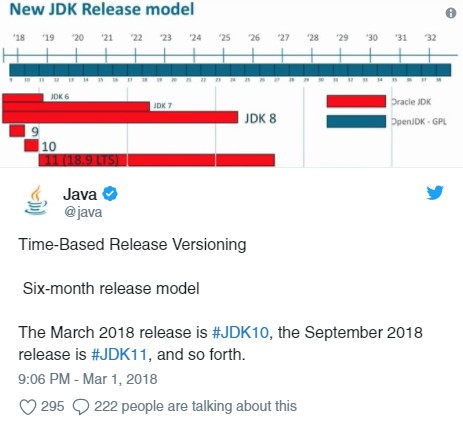
Local-Variable Type Inference
Java 10 includes a bunch of new features, but for most of us the most apparent feature is JEP 286: Local-Variable Type Inference This feature improves the developer experience by removing the boilerplate, necessary until now, to declare local variables expressing the full type fo the variable.
var shouldBeString = "Hello Java 10";
assertTrue(String.class.isInstance(shouldBeString));
From now on, local variables can be declared using the reserved word var
, type safety for the variable will be preserved by inferring the variable when its value is first assigned.
So the following code will throw a compilation failure error:
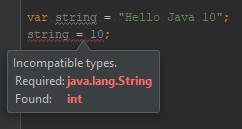
Other features included in this release
- JEP 296: Consolidate the JDK Forest into a Single Repository
- JEP 304: Garbage-Collector Interface
- JEP 307: Parallel Full GC for G1
- JEP 310: Application Class-Data Sharing
- JEP 312: Thread-Local Handshakes
- JEP 313: Remove the Native-Header Generation Tool (javah)
- JEP 314: Additional Unicode Language-Tag Extensions
- JEP 316: Heap Allocation on Alternative Memory Devices
- JEP 317: Experimental Java-Based JIT Compiler
- JEP 319: Root Certificates
Enhancements in this release
Optional.orElseThrow() Method
An additional orElseThrow()
method has been added to the Optional
class to replace the standard get()
method as the name is confusing because developers won’t expect a getter to throw an exception.
From now on this is the preferred alternative and the get()
method will be deprecated in future releases.
final Optional<String> optional = Optional.ofNullable("Optional improvement in Java 10");
final String string = optional.orElseThrow(); // Returns OK
final Optional<String> optional = Optional.ofNullable(null);
final String string = optional.orElseThrow(); // Throws NoSuchElementException
Unmodifiable collectors
New methods toUnmodifiableList()
, toUnmodifiableSet()
, and toUnmodifiableMap()
have been added to the Collectors
class to facilitate the creation of unmodifiable collections.
Stream.of(1, 2, 3).collect(Collectors.toUnmodifiableSet());
Stream.of(1, 2, 3).collect(Collectors.toUnmodifiableList());
Stream.of(1, 2, 3).collect(Collectors.toUnmodifiableMap(Function.identity(), Function.identity()));
Source code
You can find the full source code for this article with tests for the new features and enhancements at GitHub.
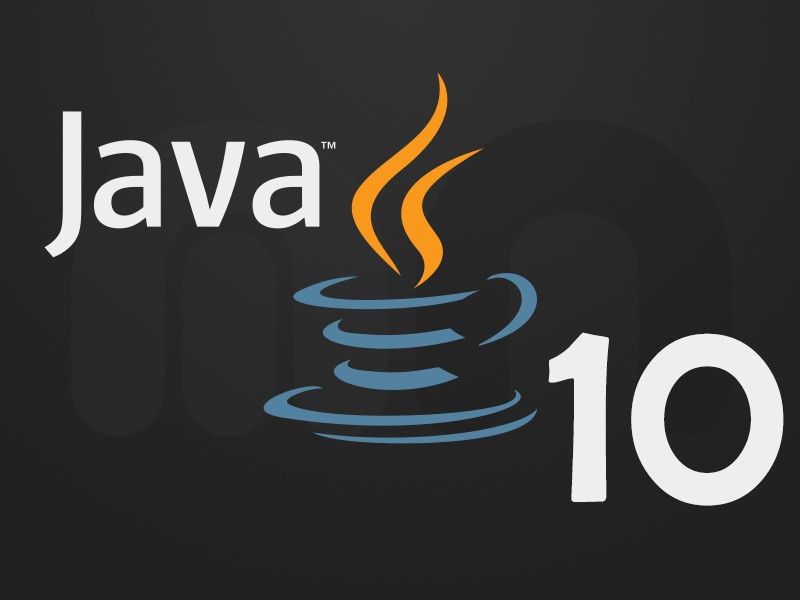