Ahoy, Helm Java!
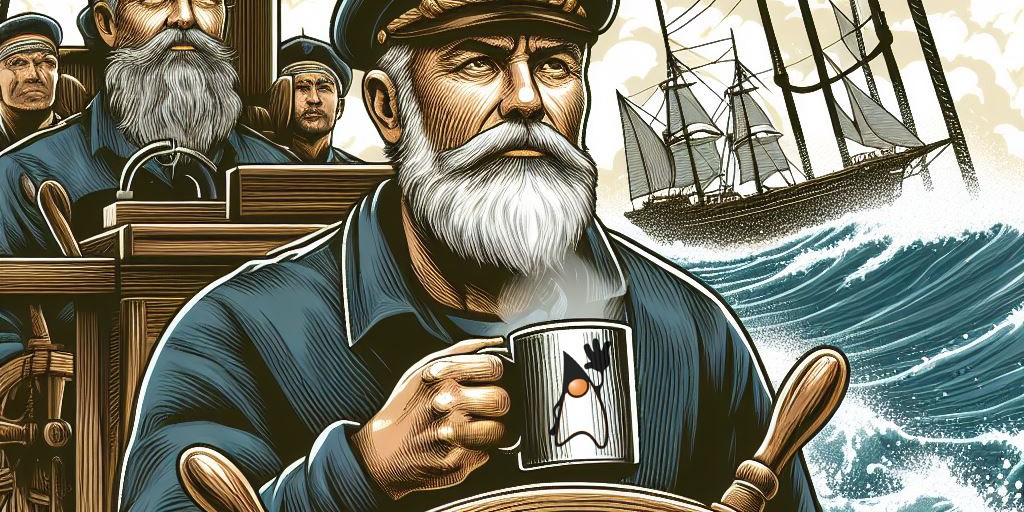
Introduction
When working with Kubernetes, Helm has become one of the go-to tools for managing the deployment of applications. Helm serves as a package manager for Kubernetes, facilitating the definition, installation, and upgrading of even the most complex Kubernetes applications.
The Helm command-line interface (CLI) is the primary tool for managing Helm charts. Typically, users invoke Helm CLI commands to create, package, install, upgrade, and roll back application releases.
While CLIs are powerful, invoking Helm commands directly from your Java application may not always be the most efficient approach. This is where Helm Java comes into play.
Helm Java is a Java library that provides a fluent API for interacting with Helm. Under the hood, Helm Java utilizes native bindings to the Helm Go library, so it behaves exactly as the Helm CLI.
Getting started
To incorporate Helm Java into your project, simply add the following dependency to your pom.xml
in case you're using Maven:
<dependency>
<groupId>com.marcnuri.helm-java</groupId>
<artifactId>helm-java</artifactId>
<version>0.0.15</version>
</dependency>
For Gradle projects, add the following to your build.gradle:
implementation 'com.marcnuri.helm-java:helm-java:0.0.15'
That's it, you're now ready to run Helm from Java!
Features
The project aims to achieve feature parity with the Helm CLI. You can check the full list of supported commands in the Helm Java GitHub repository README.md.
Currently, most of the standard Helm commands are supported, including (among many more):
- install: Install a chart.
- upgrade: Upgrade a release to a new version of a chart.
- uninstall: Uninstall a release.
- test: Run the tests for a release.
- push: Push a chart package to a chart registry.
- dependency management: Manage a chart's dependencies.
- repo management: Add, list, remove, update, and index chart repositories.
- registry: Log in or out of a Helm registry.
- lint: Check a chart for possible issues.
- package: Package a chart into a versioned archive file.
- template: Render chart templates locally.
Example Helm install
Suppose you want to install a Helm chart from a repository. You can achieve this with the following code:
import com.marcnuri.helm.Helm;
public static void main(String[] args) {
Helm.install("prometheus-community/prometheus")
.withName("my-prometheus")
.call();
}
Alternatively, you may prefer to install a chart from a local directory:
public static void main(String[] args) {
new Helm(Paths.get("path", "to", "chart"))
.install()
.withName("my-chart")
.call();
}
Most of the installation command flags are supported too:
public static void main(String[] args) {
new Helm(Paths.get("path", "to", "chart"))
.install()
.generateName()
.dependencyUpdate()
.set("value.key", "value")
.set("value.key.typed", 123)
.call();
}
As you can see, helm-java provides a fluent API that allows you to interact with Helm in a very intuitive way.
Conclusion
In this post, I've introduced you to Helm Java, a great library that allows you to interact with Helm from your Java applications. If you're considering automating Helm operations while using Java, this library is an excellent choice that will likely meet your needs while saving you valuable time.
Please check it out and let me know what you think!