Dynamic icons for your JComponents // Create an icon JButton with dynamic icons
The Internet offers countless opportunities and possibilities for developers with imagination. Today, most search engines and specialized sites offer APIs to access their data from other software, sites, devices, and so on.
In this post, I'm going to show you a dirty example of how this APIs can help you develop applications.
In less than ten lines of code (someone smarter could have done it with even less) I will add an Icon to a JButton
with an image stored on the Internet.
To accomplish this I will use Yahoo's Image Search API, documentation can be found here.
For demonstration purposes, I will use the YahooDemo applicationId
, you will need to create one if you intend to use this API in your applications.
In a nutshell, what the program does is decode what the browser gets when it reads a URL built with the API guidelines.
The response is an XML InputStream
where you can get the URL of an image thumbnail which you can then pass to the JButton
.
try {
URL url = new URL("http://search.yahooapis.com/ImageSearchService/V1/imageSearch" +
"?appid=YahooDemo&query=client%20icon&results=1&format=gif");
BufferedReader br = new BufferedReader(new InputStreamReader(url.openStream()));
String text ="";
String line = null;
while((line = br.readLine()) != null){
text += line+"\n";
}
text = text.substring(text.indexOf("<Thumbnail>"));
text = text.substring(text.indexOf("<Url>") + 5, text.indexOf("</Url>"));
BufferedImage img = ImageIO.read(new URL(text));
jButton1.setIcon(new ImageIcon(img));
} catch (MalformedURLException ex) {
ex.printStackTrace();
} catch (IOException ex) {
ex.printStackTrace();
}
Execute the code and you should get something like this:
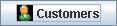
As you can see the program is quite simple.
- In the first part, we craft a URL with the desired variables.
- Next, we get the contents of the URL and parse it to get the location of the thumbnail image.
- Finally, we get the image thumbnail using
ImageIO
.
You can download a running version of the code here